Stage two of my further adventures in MIDI land. Using a single button to play a single note via MIDI. A MIDI.sendnoteon turns on a note which keeps playing unless otherwise instructed. To stop the note a second instruction is sent. MIDI.sendnoteoff
To do this you need to send a MIDI message whenever the state of the key changes. This is known as edge detection.
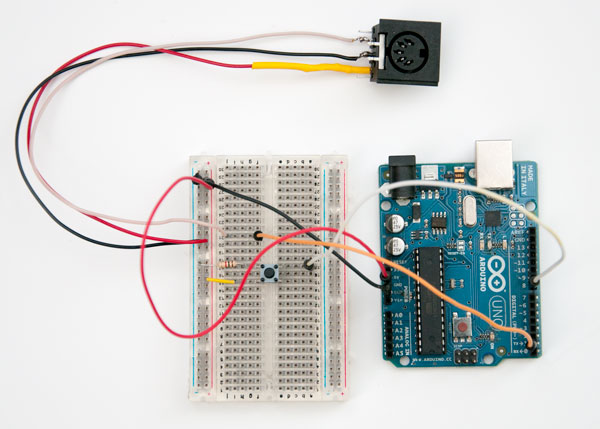
The switch in the centre of the breadboard acts as the key.
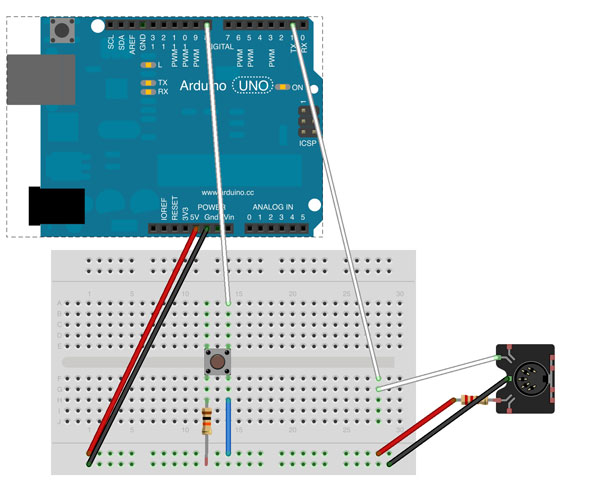
A 10k pull down resistor ensures that pin 8 on the Arduino board is kept low until the key is pressed.
// SingleButtonMIDI.ino
// Driving MIDI using a single button
// Rob Ives 2012
// This code is released into the Public Domain.
#include <MIDI.h>
#define KEY 8
#define LED 13
int keyispressed = 0; //Variable. Is the key currently pressed?
int noteisplaying = 0; //Variable. Is the Note currently playing?
void setup() //The Setup Loop
{
pinMode(LED, OUTPUT); //Set pin 13 , the led, to output
pinMode(KEY, INPUT); //Set pin 8 to input to detect the key press
MIDI.begin(); //initialise midi library
}
//---------------------------------------------
void loop() //the main loop
{
keyispressed = digitalRead(KEY); //read pin 8
if (keyispressed == HIGH){ //the key on the board is pressed
digitalWrite(LED, HIGH); //set the LED to on.
if(!noteisplaying){ //if the note is not already playing send MIDI instruction to start
MIDI.sendNoteOn(36,127,1); // Send a Note (pitch 36 (C2), vel.127 ch. 1)
noteisplaying = 1; // set the note playing flag to TRUE
}
}
else{
digitalWrite(LED,LOW); // the key is not pressed. Turn off the LED
if(noteisplaying){ //if the note is currently playing, turn it off
MIDI.sendNoteOff(36,0,1); // Stop the note
noteisplaying = 0; // clear the note is playing flag
}
}
}
Wire up the Arduino as shown and upload the Sketch. The unit will play a low C whenever the key is pressed. Works a treat for me even without switch de-bounce.